答:
using System.Text;
StringBuilder sb = new StringBuilder(0, 10000);
string strABC = “a,b,c,d,e,f,g,h,i,j,k,l,m,n,o,p,q,r,s,t,u,v,w,x,y,z”;
string[] ABC = strABC.Split(‘,’);
int len = ABC.Length;
Random rd = new Random();
for (int i = 0; i < 10000; i++)
{
sb.Append(ABC[rd.Next(len)]);
}
5.产生一个int数组,长度为100,并向其中随机插入1-100,并且不能重复。
int[] intArr=new int[100];
ArrayList myList=new ArrayList();
Random rnd=new Random();
while(myList.Count<100)
{
int num=rnd.Next(1,101);
if(!myList.Contains(num))
myList.Add(num);
}
for(int i=0;i<100;i++)
intArr[i]=(int)myList[i];
2.如何把一个Array复制到ArrayList里
答:
foreach( object o in array )arrayList.Add(o);
1. 用C#写一段选择排序算法,要求用自己的编程风格。
答:private int min;
public void xuanZhe(int[] list)//选择排序
{
for (int i = 0; i < list.Length – 1; i++)
{
min = i;
for (int j = i + 1; j < list.Length; j++)
{
if (list[j] < list[min])
min = j;
}
int t = list[min];
list[min] = list[i];
list[i] = t;
}
}
4.写一个函数计算当参数为N的值:1-2+3-4+5-6+7……+N
答:public int returnSum(int n)
{
int sum = 0;
for (int i = 1; i <= n; i++)
{
int k = i;
if (i % 2 == 0)
{
k = -k;
}
sum = sum + k;
}
return sum;
}
public int returnSum1(int n)
{
int k = n;
if (n == 0)
{
return 0;
}
if (n % 2 == 0)
{
k = -k;
}
return aaa(n – 1) + k;
}
7. 某一密码仅使用K、L、M、N、O共5个字母,密码中的单词从左向右排列,密码单词必须遵循如下规则 :
(1) 密码单词的最小长度是两个字母,可以相同,也可以不同
(2) K不可能是单词的第一个字母
(3) 如果L出现,则出现次数不止一次
(4) M不能使最后一个也不能是倒数第二个字母
(5) K出现,则N就一定出现
(6) O如果是最后一个字母,则L一定出现
问题一:下列哪一个字母可以放在LO中的O后面,形成一个3个字母的密码单词?
A) K B)L C) M D) N
答案:B
问题二:如果能得到的字母是K、L、M,那么能够形成的两个字母长的密码单词的总数是多少?
A)1个 B)3个 C)6个 D)9个
答案:A
问题三:下列哪一个是单词密码?
A) KLLN B) LOML C) MLLO D)NMKO
答案:C
62-63=1 等式不成立,请移动一个数字(不可以移动减号和等于号),使得等式成立,如何移动?
答案:62移动成2的6次方
17、列出常用的使用javascript操作xml的类包
答:
XML.prototype.xmlDoc = new ActiveXObject(“Microsoft.XMLDOM”);
XML.prototype.InitXML=InitXML;
XML.prototype.getFirstChild=getFirstChild;
XML.prototype.getLastChild=getLastChild;
XML.prototype.getChild=getChild; // 取得节点值
XML.prototype.getNodeslength=getNodeslength; // 最得节点下的子节点的个数
XML.prototype.getNode=getNode; // 取得指定节点
XML.prototype.delNode=delNode; // 删除指定节点,如果节点相同,则删除最前面的节点.
XML.prototype.getNodeAttrib=getNodeAttrib; // 取得节点的指定属性值.
XML.prototype.InsertBeforeChild=InsertBeforeChild; // 在指定节点之前插入一个节点.
XML.prototype.InsertChild=InsertChild; // 在指定节点下插入节点.
XML.prototype.setAttrib=setAttrib; // 设置指定属性的值.
XML.prototype.setNodeValue=setNodeValue; // 设置指定节点的值.
XML.prototype.CreateNodeS=CreateNodeS; // 创建一个指定名的节点.
XML.prototype.addAttrib=addAttrib; // 为指定节点添加指定属性,并设置初值.
XML.prototype.FindString=FindString; // 在指定节点下查找字符串.
给定以下XML文件,完成算法流程图<FileSystem>
< DriverC >
<Dir DirName=”MSDOS622”>
<File FileName =” Command.com” ></File>
</Dir>
<File FileName =”MSDOS.SYS” ></File>
<File FileName =” IO.SYS” ></File>
</DriverC>
</FileSystem>
请画出遍历所有文件名(FileName)的流程图(请使用递归算法)。
答:
void FindFile( Directory d )
{
FileOrFolders = d.GetFileOrFolders();
foreach( FileOrFolder fof in FileOrFolders )
{
if( fof is File )
You Found a file;
else if ( fof is Directory )
FindFile( fof );
}
}
6.C#代码实现,确保windows程序只有一个实例(instance)
///<summary>
///应用程序的主入口点。
///</summary>
[STAThread]
staticvoid Main()
{
//防止程序多次运行
if(!OneInstance.IsFirst(“GetPayInfo”))
{
MessageBox.Show (“警告:程序正在运行中! 请不要重复打开程序!可在右下角系统栏找到!”,”程序错误提
示:”,MessageBoxButtons.OK,MessageBoxIcon.Stop);
return;
}
Application.Run(new Form1());
}
// ******************* 防止程序多次执行 **************************
publicabstractclass OneInstance
{
///<summary>
///判断程序是否正在运行
///</summary>
///<param name=”appId”>程序名称</param>
///<returns>如果程序是第一次运行返回True,否则返回False</returns>
publicstaticbool IsFirst(string appId)
{
bool ret=false;
if(OpenMutex(0x1F0001,0,appId)==IntPtr.Zero)
{
CreateMutex(IntPtr.Zero,0,appId);
ret=true;
}
return ret;
}
[DllImport("Kernel32.dll",CharSet=CharSet.Auto)]
privatestaticextern IntPtr OpenMutex(
uint dwDesiredAccess, // access
int bInheritHandle, // inheritance option
string lpName // object name
);
[DllImport("Kernel32.dll",CharSet=CharSet.Auto)]
privatestaticextern IntPtr CreateMutex(
IntPtr lpMutexAttributes, // SD
int bInitialOwner, // initial owner
string lpName // object name
);
}
6.一列数的规则如下: 1、1、2、3、5、8、13、21、34…… 求第30位数是多少, 用递归算法实现。
答:public class MainClass
{
public static void Main()
{
Console.WriteLine(Foo(30));
}
public static int Foo(int i)
{
if (i <= 0)
return 0;
else if(i > 0 && i <= 2)
return 1;
else return Foo(i -1) + Foo(i – 2);
}
}
8.请编程实现一个冒泡排序算法?
答:
int [] array = new int ;
int temp = 0 ;
for (int i = 0 ; i < array.Length – 1 ; i++)
{
for (int j = i + 1 ; j < array.Length ; j++)
{
if (array[j] < array[i])
{
temp = array[i] ;
array[i] = array[j] ;
array[j] = temp ;
}
}
}
11.在下面的例子里
using System;
class A
{
public A()
{
PrintFields();
}
public virtual void PrintFields(){}
}
class B:A
{
int x=1;
int y;
public B()
{
y=-1;
}
public override void PrintFields()
{
Console.WriteLine(“x={0},y={1}”,x,y);
}
}
当使用new B()创建B的实例时,产生什么输出?
答:X=1,Y=0;
3.下面的例子中
using System;
class A
{
public static int X;
static A(){
X=B.Y+1;
}
}
class B
{
public static int Y=A.X+1;
static B(){}
static void Main(){
Console.WriteLine(“X={0},Y={1}”,A.X,B.Y);
}
}
产生的输出结果是什么?x=1,y=2
15.根据委托(delegate)的知识,请完成以下用户控件中代码片段的填写:
namespace test
{
public delegate void OnDBOperate();
public class UserControlBase : System.Windows.Forms.UserControl
{
public event OnDBOperate OnNew;
privatevoidtoolBar_ButtonClick(objectsender,System.Windows.Forms.ToolBarButtonClickEventArgs e)
{
if(e.Button.Equals(BtnNew))
{
//请在以下补齐代码用来调用OnDBOperate委托签名的OnNew事件。
}
}
}
答:if( OnNew != null )
OnNew( this, e );
16.分析以下代码,完成填空
string strTmp = “abcdefg某某某”;
int i= System.Text.Encoding.Default.GetBytes(strTmp).Length;
int j= strTmp.Length;
以上代码执行完后,i= j=
答:i=13,j=10
17.给定以下XML文件,完成算法流程图。
<FileSystem>
< DriverC >
<Dir DirName=”MSDOS622”>
<File FileName =” Command.com” ></File>
</Dir>
<File FileName =”MSDOS.SYS” ></File>
<File FileName =” IO.SYS” ></File>
</DriverC>
</FileSystem>
请画出遍历所有文件名(FileName)的流程图(请使用递归算法)。
答:伪代码:
void FindFile( Directory d )
{
FileOrFolders = d.GetFileOrFolders();
foreach( FileOrFolder fof in FileOrFolders )
{
if( fof is File )
You Found a file;
else if ( fof is Directory )
FindFile( fof );
}
}
C#:
Public void DomDepthFirst(XmlNode currentNode)
{
XmlNode node=currentNode.FirstChild;
while(node!=null)
{
DomDepthFirst(node);
node=node.NextSibling;
}
if(node.Name==”File”)
{
Console.Write(((XmlElement)node).GetAttribute(“FileName”)+”rn”);
}
}
29.根据线程安全的相关知识,分析以下代码,当调用test方法时i>10时是否会引起死锁?并简要说明理由。
public void test(int i)
{
lock(this)
{
if (i>10)
{
i–;
test(i);
}
}
}
答:不会发生死锁,(但有一点int是按值传递的,所以每次改变的都只是一个副本,因此不会出现死锁。但如果把int换做一个object,那么死锁会发生)
78、下面的程序执行结果是什么?
class Person
{
public int Age { get; set; }
}
int i1 = 20;
int i2 = i1;
i1++;
Console.WriteLine(i2);
Person p1 = new Person();
p1.Age = 20;
Person p2 = p1;
p1.Age++;
Console.WriteLine(p2.Age);
答案:
20 、21
2、不用中间变量交换两个变量
int i = 500;
int j = int.MaxValue – 10;
//int i = 10;
//int j = 20;
Console.WriteLine(“i={0},j={1}”, i, j);
i = i + j;//i=30
j = i – j;//j=10;
i = i – j;//i=20;
Console.WriteLine(“i={0},j={1}”,i,j);
有一个10个数的数组,计算其中不重复数字的个数。{3,5,9,8,10,5,3}
用HashSet
int[] values = { 3, 5, 9, 8, 10, 5, 3 };
HashSet<int> set = new HashSet<int>();
foreach (int i in values)
{
set.Add(i);
}
foreach (int i in set)
{
Console.WriteLine(i);
}
点击table获取行号列号?
function getRowAndColumn()
{
if(!document.getElementsByTagName || !document.createTextNode)return;
varrows=document.getElementById(‘MyTable’).getElementsByTagName(‘tr’);
varcols;
for(i = 0; i < rows.length; i++)
{
rows[i].onclick=function()
{
10. alert(“行:”+eval(this.rowIndex + 1));
11. }
12. if(i=0)
13. {
14. colsTH =rows[i].getElementsByTagName(‘th’);
15. alert(colsTH.length);
16. for(k = 0; k< colsTH.length; k++)
17. {
18. colsTH[k].onclick =function()
19. {
20. alert(“列:”+eval(this.cellIndex +1));
21. }
22. }
23. }
24. else
25. {
26. cols =rows[i].getElementsByTagName(‘td’);
27. for(j = 0; j < cols.length; j++)
28. {
29. cols[j].onclick =function()
30. {
31. alert(“列:”+eval(this.cellIndex + 1));
32. }
33. }
34. }
35. }
36. }
//相传有一群猴子要选出大王,它们采用的方式为:所有猴子站成一个圈,然后从1开始报数,每当数到”.
//”N的那一只猴子就出列,然后继续从下一个猴子开始又从1开始数,数到N的猴子继续出列,一直到最后”.
//”剩的猴子就是大王了。假如现在有M只猴子,报数数为N,请问第几只猴子是大王?列出选大王的过程。
int M = 10;
int N = 3;
List<int> monkeys = new List<int>();
for (int i = 1; i <= M; i++)
{
monkeys.Add(i);
}
int currentIndex = 0;
while (true)
{
for (int i = 1; i <= N; i++)
{
if (i == N)
{
monkeys.RemoveAt(currentIndex);
if (monkeys.Count == 1)
{
Console.WriteLine(monkeys[0]);
return;
}
}
currentIndex++;
if (currentIndex >= monkeys.Count)
{
currentIndex = 0;
}
}
}
38:请编程遍历页面上所有TextBox控件并给它赋值为string.Empty?
答:
foreach (System.Windows.Forms.Control control in this.Controls)
{
if (control is System.Windows.Forms.TextBox)
{
System.Windows.Forms.TextBox tb = (System.Windows.Forms.TextBox)control ;
tb.Text = String.Empty ;
}
}
10. 程序设计: 猫大叫一声,所有的老鼠都开始逃跑,主人被惊醒。(C#语言)
要求: 1.要有联动性,老鼠和主人的行为是被动的。
2.考虑可扩展性,猫的叫声可能引起其他联动效应。
要点:1. 联动效果,运行代码只要执行Cat.Cryed()方法。2. 对老鼠和主人进行抽象
评分标准: <1>.构造出Cat、Mouse、Master三个类,并能使程序运行
<2>从Mouse和Master中提取抽象
<3>联动效应,只要执行Cat.Cryed()就可以使老鼠逃跑,主人惊醒。
public interface Observer
{
void Response(); //观察者的响应,如是老鼠见到猫的反映
}
public interface Subject
{
void AimAt(Observer obs); //针对哪些观察者,这里指猫的要扑捉的对象—老鼠
}
public class Mouse : Observer
{
private string name;
public Mouse(string name, Subject subj)
{
this.name = name;
subj.AimAt(this);
}
public void Response()
{
Console.WriteLine(name + ” attempt to escape!”);
}
}
public class Master : Observer
{
public Master(Subject subj)
{
subj.AimAt(this);
}
public void Response()
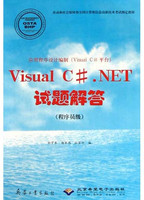
{
Console.WriteLine(“Host waken!”);
}
}
public class Cat : Subject
{
private ArrayList observers;
public Cat()
{
this.observers = new ArrayList();
}
public void AimAt(Observer obs)
{
this.observers.Add(obs);
}
public void Cry()
{
Console.WriteLine(“Cat cryed!”);
foreach (Observer obs in this.observers)
{
obs.Response();
}
}
}
class MainClass
{
static void Main(string[] args)
{
Cat cat = new Cat();
Mouse mouse1 = new Mouse(“mouse1″, cat);
Mouse mouse2 = new Mouse(“mouse2″, cat);
Master master = new Master(cat);
cat.Cry();
}
}
//———————————————————————————————
设计方法二: 使用event — delegate设计..
public delegate void SubEventHandler();
public abstract class Subject
{
public event SubEventHandler SubEvent;
protected void FireAway()
{
if (this.SubEvent != null)
this.SubEvent();
}
}
public class Cat : Subject
{
public void Cry()
{
Console.WriteLine(“cat cryed.”);
this.FireAway();
}
}
public abstract class Observer
{
public Observer(Subject sub)
{
sub.SubEvent += new SubEventHandler(Response);
}
public abstract void Response();
}
public class Mouse : Observer
{
private string name;
public Mouse(string name, Subject sub) : base(sub)
{
this.name = name;
}
public override void Response()
{
Console.WriteLine(name + ” attempt to escape!”);
}
}
public class Master : Observer
{
public Master(Subject sub) : base(sub){}
public override void Response()
{
Console.WriteLine(“host waken”);
}
}
class Class1
{
static void Main(string[] args)
{
Cat cat = new Cat();
Mouse mouse1 = new Mouse(“mouse1″, cat);
Mouse mouse2 = new Mouse(“mouse2″, cat);
Master master = new Master(cat);
cat.Cry();
}
}