Project design
Using link_list to realize the booklibary Management System
Library is a place that provide students to borrow and return.The books information is very important for students.
Now design a project to Imitate the library and can retrieve information fastly.
The books have this type of structure which are book_id ,bookname,book type,books_status.
You can define structure and program as following.
1) add books
2) list books
3) search books
4) borrow books
5) return books
6) exit
add books : you can type from keyboard to input books information one by one.
Each book should be given some prompt ,for example : “you input the 1str book id :” and so on.
List books : after you select 2,then display all books according to certain format
Search books : you can select some books according to book name or book type.So you should create sub menu to selected.
Borrow books: means borrow some books from library,so in the library the book status will be edited to having borrowed.
return books: put the books status change no having borrowed.
*/
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#define BORROWED 1
#define NOBORROW 0
/* 定义图书结构 */
struct Book
{
int _id; // 编号,唯一
char _name[32]; // 图书的名称,不唯一
int _type; // 图书的类型,不唯一
int _status; // 图书的状态,借出/没有借出
int _count; // 图书借出的次数
struct Book* _next; // 下一本书
struct Book* _prev; // 上一本书
};
/* 定义图书类型 */
typedef struct Book Book;
/* 操作的目录提示 */
char book_menu[][32] =
{
"add books",
"list books",
"search books",
"borrow books",
"return books",
"exit",
"",
};
/* 操作的目录提示1 */
char book_menu_sub[][32] =
{
"search books by name",
"search books by type",
"",
};
/* 添加图书类型,用户信息显示 */
char book_types[][32] =
{
"type0",
"type1",
"type2",
"",
};
/* 添加图书状态,用户信息显示 */
char book_status[][32] =
{
"noborrow",
"borrowed",
"",
};
/* 增加图书,id自动排号 */
int add_books(Book* head, Book* book_for_add)
{
Book* p = head;
if (!p)
return -1;
while (p->_next)
{
p = p->_next;
};
p->_next = book_for_add;
book_for_add->_id = p->_id+1;
book_for_add->_prev = p;
book_for_add->_next = NULL;
book_for_add->_count = 0;
book_for_add->_status = 0;
return 0;
};
/* 建立图书管理链表 */
Book* create_list(Book* book_head)
{
if (book_head)
{
book_head->_prev = book_head;
book_head->_next = NULL;
book_head->_id = 1;
book_head->_count = 0;
book_head->_status = 0;
}
return book_head;
}
/* 释放链表 */
void free_list(Book* book_head)
{
Book *p, *p1;
p = book_head;
while(p)
{
p1 = p->_next;
free(p);
p = p1;
}
}
/* 打印图书信息 */
void print_book(Book* book)
{
printf("id:%d, name:%s, type:%s, status:%s, times:%d n",
book->_id, book->_name, book_types[book->_type],
book_status[book->_status], book->_count);
}
/* 列出所有登记的图书 */
void list_books(Book* book_head)
{
Book* p = book_head;
while (p)
{
print_book(p);
p = p->_next;
}
}
/* 借书,返回该书的指针,否则就返回NULL,可能不存在图书,或者已经被借走了 */
Book* borrow_books(Book* book_head, int id)
{
Book* p = book_head;
while (p)
{
if (p->_id == id)
break;
p = p->_next;
}
if (!p)/* 不存在 */
return NULL;
if (p->_status != 0) /* 已经被借走 */
return NULL;
p->_status = 1; /* 借书登记 */
p->_count++; /* 被借阅次数+1 */
return p;
}
/* 查找书名称,返回查找到的数量,书名可能有重复的,一本书可能也有很多本,但是ID却是唯一的 */
int search_books_by_name(Book* book_head, char* name)
{
int count = 0;
Book* p = book_head;
while (p)
{
if (strcmp(p->_name, name) == 0)
{
print_book(p);
++count;
}
p = p->_next;
}
return count;
}
/* 查找书类型,返回查找到的数量,一个类型会有很多书 */
int search_books_by_type(Book* book_head, int type)
{
int count = 0;
Book* p = book_head;
while (p)
{
if (p->_type == type)
{
print_book(p);
++count;
}
p = p->_next;
}
return count;
}
/* 还书,正常归还就返回该书的指针,否则就返回NULL */
Book* return_books(Book* book_head, int id)
{
Book* p = book_head;
while (p)
{
if (p->_id == id && p->_status != 0)
{
p->_status = 0; /* 还书登记 */
break;
}
p = p->_next;
}
return p;
}
/* 打印操作选项 */
void print_menu(char menus[][32])
{
int no = 0;
printf("nPLEASE SELECT ID LIKE 1 or 2 ..n");
while (strlen(menus[no]))
{
printf("%d t%sn", no+1, menus[no]);
++no;
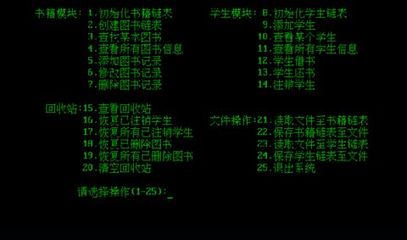
}
printf("OPTION(");
while (no)
{
printf("/%d", no--);
}
printf("):");
}
/* 主函数 */
int main(void)
{
char key = 0;
char ss[128];
int loop = 1;
Book first_book, *pBooks, *pTmp;
strcpy(first_book._name, "nihao");
first_book._type = 0;
pBooks = create_list(&first_book);
while (loop)
{
print_menu(book_menu);
gets(ss);
key = ss[0] - 48;
if(key < 0 || key > 6)
printf("Error input !n");
else
printf("INPUT:%dn", key);
switch (key)
{
case 1: // add books
pTmp = (Book*)malloc(sizeof(Book));
if(pTmp)
{
printf("Add_Book input name:");
gets(ss);
strncpy(pTmp->_name, ss, 31);
print_menu(book_types);
gets(ss);
key = ss[0] - 48;
if(key <= 0 || key > (sizeof(book_types)/32) )
key = 1;
pTmp->_type = key - 1;
add_books(pBooks, pTmp);
}
break;
case 2: // list books
list_books(pBooks);
break;
case 3: // search books
print_menu(book_menu_sub);
gets(ss);
key = ss[0] - 48;
printf("INPUT:%dn", key);
switch (key)
{
case 1: // search books by name
printf("input book name:");
gets(ss);
key = search_books_by_name(pBooks, ss);
if(key == 0)
printf("no found!n");
case 2: // search books by type
print_menu(book_types);
gets(ss);
key = ss[0] - 48;
key = search_books_by_type(pBooks, key-1);
if(key == 0)
printf("no found!n");
default:
break;
}
break;
case 4: // borrow books
printf("Borrow books, input book id:");
gets(ss);
key = atoi(ss);
pTmp = borrow_books(pBooks, key);
if (!pTmp)
printf("borrow books failed: %dn", key);
else
{
printf("borrow books successful!n");
print_book(pTmp);
}
break;
case 5: // return books
printf("Return books, input book id:");
gets(ss);
key = atoi(ss);
pTmp = return_books(pBooks, key);
if (!pTmp)
printf("return books failed: %dn", key);
else
{
printf("return books successful!n");
print_book(pTmp);
}
break;
case 6: // exit
loop = 0;
break;
default:
break;
}
printf("--pause--");
gets(ss);
}
free_list(pBooks);
return 0;
}